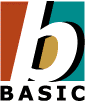 |
Tutorial 06: Reading and Writing Data
September 20, 2009
© NSB Corporation. All rights reserved.
Contributed by Tom Newman, Mission Peak Designs
|
Contents:
- Introduction
- Getting Started
- Creating the Form
- Creating the Code
- Writing String Data
- Reading String Data
- Testing the Program
Introduction
The purpose of this tutorial is to demonstrate reading and writing data
to a file using NS Basic/CE. You should have completed Tutorial #1
before beginning this tutorial.
The program to be developed uses NewObject's ActiveX Storage and
File control to create, write, and read files. This tutorial will create
a program that writes and reads string data to a file.
Getting Started
Including file reading/writing support in your application starts as simply as adding NewObjects ActiveX control as a resource to your program. Select Project > Add Resource, then select newObjectsPack1.dll.
(For a complete reference on the methods above, make sure you read the
Tech Note 08: File I/O Control - SFStream and
Tech Note 08a: File I/O Control with Fields - SFRecord)
Creating the Form
Start a new "Standard" project and save it as FileIO.nsb
Create the following controls on the form:
- Create a CommandButton and call it "cmdCreate". Change the Caption
to "Create a File".
- Create a CommandButton and call it "cmdWrite". Change the Caption
to "Write 1000 Records".
- Create a CommandButton and call it "cmdRead". Change the Caption
to "Read 1000 Records".
- Create a CommandButton and call it "cmdRandomRead". Change the Caption
to "Do 1000 Random".
- Create a CommandButton and call it "cmdDelete". Change the Caption
to "Delete a File".
- Create a TextBox and call it "txtMsg". Change Multiline to True.
Change Scrollbars to 2 (vertical scrollbars). Delete the
field after the Text property.
The form should look like the following:
Creating the Code
First off we will create code that initializes our program and creates
and deletes our test file.
Enter the following code in the Code Window (you can copy and paste
this code):
Now let's analyze the code, line by line:
- Line 8 is the Form1_Load routine and is called after the form and
controls have been created.
- Line 9 creates our Storage and File object (SFMain).
- Line 13 is the cmdCreate_Click routine called from the "Create a File"
button. This routine creates our test file if it doesn't already exist.
- Line 24 is the cmdDelete_Click routine called from the "Delete a File"
button. This routine deletes our test file if it exists.
Writing String Data
Next we will create code that writes 1000 records (strings) to
our test file.
Now let's analyze the code, line by line:
- Line 35 is the cmdWrite_Click routine called from the "Write 1000
Records" button.
- Line 38 verifies that the file exists.
- Line 43 opens the file.
- Line 45 uses WriteText to write one string to the file. (WriteText
writes the end of line character at the end of the write.)
- Line 49 closes the file after writing 1000 strings.
Reading String Data
Next we will create code that reads 1000 records (strings) from
our test file and follow that up by code that reads 1000 random records.
Now let's analyze the code, line by line:
- Line 52 is the cmdRead_Click routine called from the "Read 1000
Records" button.
- Line 55 verifies that the file exists.
- Line 60 opens the file.
- Line 63 uses ReadText to read one record (string) from the file.
(ReadText reads up until the end of line character is found.)
- Line 65 loops reading the file until End of File (EOF) is found.
- Line 68 closes the file.
- Line 71 is the cmdRandom_Click routine called from the "Do 1000
Random" button.
- Line 74 verifies that the file exists.
- Line 79 opens the file.
- Line 80 gets the size of the file.
- Line 82 computes a random position in the file and uses Pos to set
the file pointer to that position.
- Line 83 uses ReadText to read one record (string) from the file
(starting at the random position).
- Line 84 loops until 1000 random reads have occurred.
- Line 87 closes the file.
Testing the Program
Press F5 to save and start the program. Your results should look similar
to the image below. The program creates a file (MyFile.txt) and writes/reads
1000 strings. The last button deletes the file.